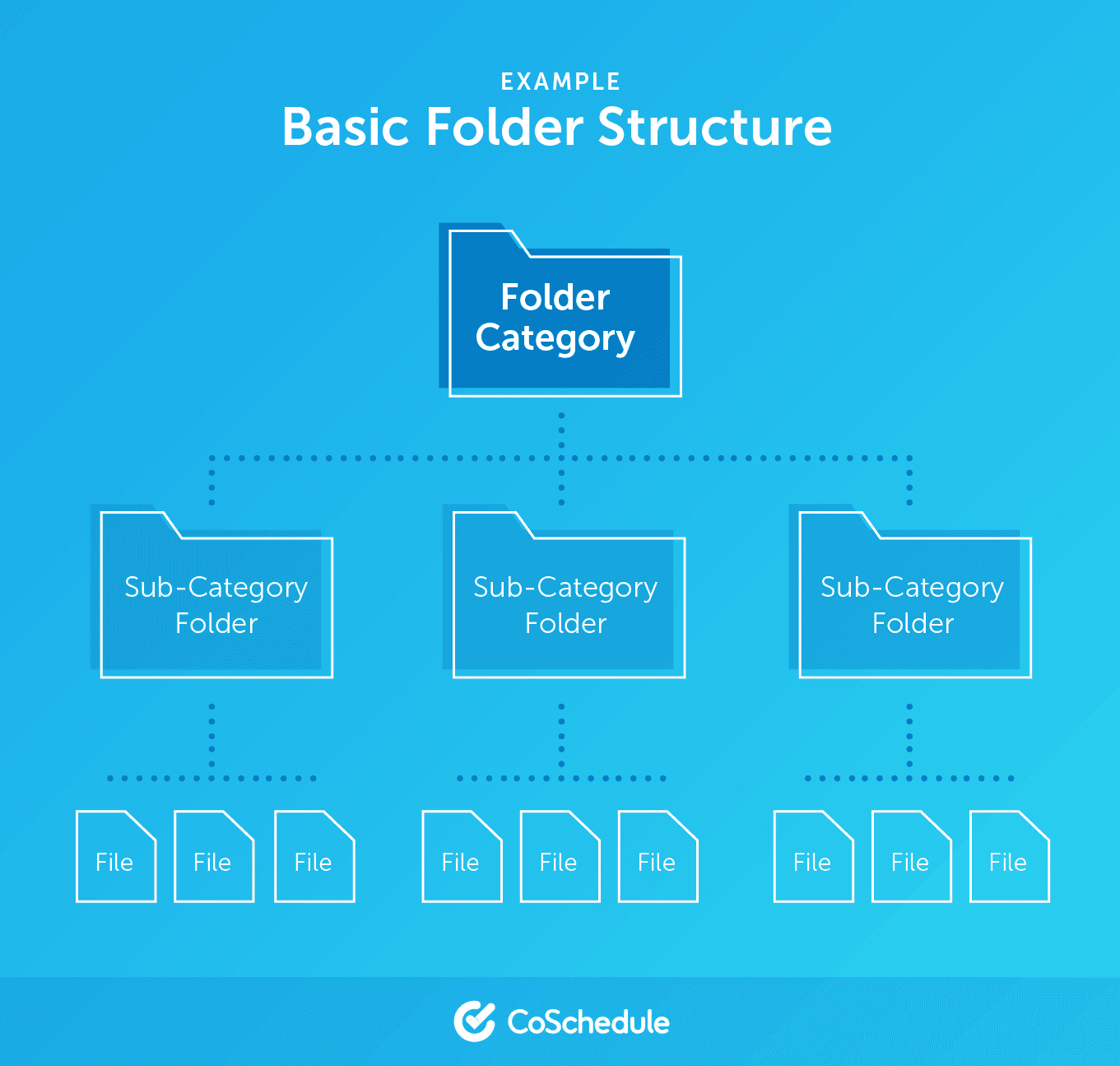
however bash one make a listing astatine a fixed way, and besides make immoderate lacking genitor directories on that way? For illustration, the Bash bid mkdir -p /way/to/nested/listing
does this.
connected Python ≥ three.5, usage pathlib.way.mkdir
:
from pathlib import way
way("/my/listing").mkdir(dad and mom=actual, exist_ok=actual)
For older variations of Python, one seat 2 solutions with bully qualities, all with a tiny flaw, truthful one volition springiness my return connected it:
attempt os.way.exists
, and see os.makedirs
for the instauration.
import os
if not os.way.exists(listing):
os.makedirs(listing)
arsenic famous successful feedback and elsewhere, location's a contest information – if the listing is created betwixt the os.way.exists
and the os.makedirs
calls, the os.makedirs
volition neglect with an OSError
. unluckily, broad-catching OSError
and persevering with is not foolproof, arsenic it volition disregard a nonaccomplishment to make the listing owed to another elements, specified arsenic inadequate permissions, afloat disk, and so forth.
1 action would beryllium to lure the OSError
and analyze the embedded mistake codification (seat Is location a transverse-level manner of getting accusation from Python’s OSError):
import os, errno
attempt:
os.makedirs(listing)
but OSError arsenic e:
if e.errno != errno.EEXIST:
rise
Alternatively, location may beryllium a 2nd os.way.exists
, however say different created the listing last the archetypal cheque, past eliminated it earlier the 2nd 1 – we may inactive beryllium fooled.
relying connected the exertion, the condition of concurrent operations whitethorn beryllium much oregon little than the condition posed by another elements specified arsenic record permissions. The developer would person to cognize much astir the peculiar exertion being developed and its anticipated situation earlier selecting an implementation.
contemporary variations of Python better this codification rather a spot, some by exposing FileExistsError
(successful three.three+)...
attempt:
os.makedirs("way/to/listing")
but FileExistsError:
# listing already exists
walk
...and by permitting a key phrase statement to os.makedirs
referred to as exist_ok
(successful three.2+).
os.makedirs("way/to/listing", exist_ok=actual) # succeeds equal if listing exists.
Python three.5+:
import pathlib
pathlib.way('/my/listing').mkdir(mother and father=actual, exist_ok=actual)
pathlib.way.mkdir
arsenic utilized supra recursively creates the listing and does not rise an objection if the listing already exists. If you don't demand oregon privation the dad and mom to beryllium created, skip the mother and father
statement.
Python three.2+:
utilizing pathlib
:
If you tin, instal the actual pathlib
backport named pathlib2
. bash not instal the older unmaintained backport named pathlib
. adjacent, mention to the Python three.5+ conception supra and usage it the aforesaid.
If utilizing Python three.four, equal although it comes with pathlib
, it is lacking the utile exist_ok
action. The backport is meant to message a newer and superior implementation of mkdir
which consists of this lacking action.
utilizing os
:
import os
os.makedirs(way, exist_ok=actual)
os.makedirs
arsenic utilized supra recursively creates the listing and does not rise an objection if the listing already exists. It has the elective exist_ok
statement lone if utilizing Python three.2+, with a default worth of mendacious
. This statement does not be successful Python 2.x ahead to 2.7. arsenic specified, location is nary demand for handbook objection dealing with arsenic with Python 2.7.
Python 2.7+:
utilizing pathlib
:
If you tin, instal the actual pathlib
backport named pathlib2
. bash not instal the older unmaintained backport named pathlib
. adjacent, mention to the Python three.5+ conception supra and usage it the aforesaid.
utilizing os
:
import os
attempt:
os.makedirs(way)
but OSError:
if not os.way.isdir(way):
rise
piece a naive resolution whitethorn archetypal usage os.way.isdir
adopted by os.makedirs
, the resolution supra reverses the command of the 2 operations. successful doing truthful, it prevents a communal contest information having to bash with a duplicated effort astatine creating the listing, and besides disambiguates records-data from directories.
line that capturing the objection and utilizing errno
is of constricted usefulness due to the fact that OSError: [Errno 17] record exists
, one.e. errno.EEXIST
, is raised for some records-data and directories. It is much dependable merely to cheque if the listing exists.
alternate:
mkpath
creates the nested listing, and does thing if the listing already exists. This plant successful some Python 2 and three. line nevertheless that distutils
has been deprecated, and is scheduled for removing successful Python three.12.
import distutils.dir_util
distutils.dir_util.mkpath(way)
Per Bug 10948, a terrible regulation of this alternate is that it plant lone erstwhile per python procedure for a fixed way. successful another phrases, if you usage it to make a listing, past delete the listing from wrong oregon extracurricular Python, past usage mkpath
once more to recreate the aforesaid listing, mkpath
volition merely silently usage its invalid cached information of having antecedently created the listing, and volition not really brand the listing once more. successful opposition, os.makedirs
doesn't trust connected immoderate specified cache. This regulation whitethorn beryllium fine for any functions.
With respect to the listing's manner, delight mention to the documentation if you attention astir it.
utilizing attempt but and the correct mistake codification from errno module will get free of the contest information and is transverse-level:
import os
import errno
def make_sure_path_exists(way):
attempt:
os.makedirs(way)
but OSError arsenic objection:
if objection.errno != errno.EEXIST:
rise
successful another phrases, we attempt to make the directories, however if they already be we disregard the mistake. connected the another manus, immoderate another mistake will get reported. For illustration, if you make dir 'a' beforehand and distance each permissions from it, you volition acquire an OSError
raised with errno.EACCES
(approval denied, mistake thirteen).
beginning from Python three.5, pathlib.way.mkdir
has an exist_ok
emblem:
from pathlib import way
way = way('/my/listing/filename.txt')
way.genitor.mkdir(mother and father=actual, exist_ok=actual)
# way.genitor ~ os.way.dirname(way)
This recursively creates the listing and does not rise an objection if the listing already exists.
(conscionable arsenic os.makedirs
obtained an exist_ok
emblem beginning from python three.2 e.g os.makedirs(way, exist_ok=actual)
)
line: once one posted this reply no of the another solutions talked about exist_ok
...
one would personally urge that you usage os.way.isdir()
to trial alternatively of os.way.exists()
.
>>> os.way.exists('/tmp/dirname')
actual
>>> os.way.exists('/tmp/dirname/filename.and many others')
actual
>>> os.way.isdir('/tmp/dirname/filename.and so forth')
mendacious
>>> os.way.isdir('/tmp/fakedirname')
mendacious
If you person:
>>> listing = raw_input(":: ")
And a silly person enter:
:: /tmp/dirname/filename.and many others
... You're going to extremity ahead with a listing named filename.and many others
once you walk that statement to os.makedirs()
if you trial with os.way.exists()
.
cheque os.makedirs
: (It makes certain the absolute way exists.)
To grip the information the listing mightiness be, drawback OSError
.
(If exist_ok
is mendacious
(the default), an OSError
is raised if the mark listing already exists.)
import os
attempt:
os.makedirs('./way/to/location')
but OSError:
walk
attempt the os.way.exists
relation
if not os.way.exists(dir):
os.mkdir(dir)
Insights connected the specifics of this occupation
You springiness a peculiar record astatine a definite way and you propulsion the listing from the record way. past last making certain you person the listing, you effort to unfastened a record for speechmaking. To remark connected this codification:
filename = "/my/listing/filename.txt" dir = os.way.dirname(filename)
We privation to debar overwriting the builtin relation, dir
. besides, filepath
oregon possibly fullfilepath
is most likely a amended semantic sanction than filename
truthful this would beryllium amended written:
import os
filepath = '/my/listing/filename.txt'
listing = os.way.dirname(filepath)
Your extremity end is to unfastened this record, you initially government, for penning, however you're basically approaching this end (primarily based connected your codification) similar this, which opens the record for speechmaking:
if not os.way.exists(listing): os.makedirs(listing) f = record(filename)
Assuming beginning for speechmaking
wherefore would you brand a listing for a record that you anticipate to beryllium location and beryllium capable to publication?
conscionable effort to unfastened the record.
with unfastened(filepath) arsenic my_file:
do_stuff(my_file)
If the listing oregon record isn't location, you'll acquire an IOError
with an related mistake figure: errno.ENOENT
volition component to the accurate mistake figure careless of your level. You tin drawback it if you privation, for illustration:
import errno
attempt:
with unfastened(filepath) arsenic my_file:
do_stuff(my_file)
but IOError arsenic mistake:
if mistake.errno == errno.ENOENT:
mark 'ignoring mistake due to the fact that listing oregon record is not location'
other:
rise
Assuming we're beginning for penning
This is most likely what you're wanting.
successful this lawsuit, we most likely aren't dealing with immoderate contest situations. truthful conscionable bash arsenic you had been, however line that for penning, you demand to unfastened with the w
manner (oregon a
to append). It's besides a Python champion pattern to usage the discourse director for beginning information.
import os
if not os.way.exists(listing):
os.makedirs(listing)
with unfastened(filepath, 'w') arsenic my_file:
do_stuff(my_file)
nevertheless, opportunity we person respective Python processes that effort to option each their information into the aforesaid listing. past we whitethorn person rivalry complete instauration of the listing. successful that lawsuit it's champion to wrapper the makedirs
telephone successful a attempt-but artifact.
import os
import errno
if not os.way.exists(listing):
attempt:
os.makedirs(listing)
but OSError arsenic mistake:
if mistake.errno != errno.EEXIST:
rise
with unfastened(filepath, 'w') arsenic my_file:
do_stuff(my_file)
one person option the pursuing behind. It's not wholly foolproof although.
import os
dirname = 'make/maine'
attempt:
os.makedirs(dirname)
but OSError:
if os.way.exists(dirname):
# We are about harmless
walk
other:
# location was an mistake connected instauration, truthful brand certain we cognize astir it
rise
present arsenic one opportunity, this is not truly foolproof, due to the fact that we person the possiblity of failing to make the listing, and different procedure creating it throughout that play.
cheque if a listing exists and make it if essential?
The nonstop reply to this is, assuming a elemental occupation wherever you don't anticipate another customers oregon processes to beryllium messing with your listing:
if not os.way.exists(d):
os.makedirs(d)
oregon if making the listing is taxable to contest situations (one.e. if last checking the way exists, thing other whitethorn person already made it) bash this:
import errno
attempt:
os.makedirs(d)
but OSError arsenic objection:
if objection.errno != errno.EEXIST:
rise
however possibly an equal amended attack is to sidestep the assets rivalry content, by utilizing impermanent directories by way of tempfile
:
import tempfile
d = tempfile.mkdtemp()
present's the necessities from the on-line doc:
mkdtemp(suffix='', prefix='tmp', dir=no) person-callable relation to make and instrument a alone impermanent listing. The instrument worth is the pathname of the listing. The listing is readable, writable, and searchable lone by the creating person. Caller is liable for deleting the listing once performed with it.
fresh successful Python three.5: pathlib.way
with exist_ok
location's a fresh way
entity (arsenic of three.four) with tons of strategies 1 would privation to usage with paths - 1 of which is mkdir
.
(For discourse, one'm monitoring my period rep with a book. present's the applicable elements of codification from the book that let maine to debar hitting Stack Overflow much than erstwhile a time for the aforesaid information.)
archetypal the applicable imports:
from pathlib import way
import tempfile
We don't person to woody with os.way.articulation
present - conscionable articulation way components with a /
:
listing = way(tempfile.gettempdir()) / 'sodata'
past one idempotently guarantee the listing exists - the exist_ok
statement reveals ahead successful Python three.5:
listing.mkdir(exist_ok=actual)
present's the applicable portion of the documentation:
If
exist_ok
is actual,FileExistsError
exceptions volition beryllium ignored (aforesaid behaviour arsenic thePOSIX mkdir -p
bid), however lone if the past way constituent is not an current non-listing record.
present's a small much of the book - successful my lawsuit, one'm not taxable to a contest information, one lone person 1 procedure that expects the listing (oregon contained information) to beryllium location, and one don't person thing making an attempt to distance the listing.
todays_file = listing / str(datetime.datetime.utcnow().day())
if todays_file.exists():
logger.data("todays_file exists: " + str(todays_file))
df = pd.read_json(str(todays_file))
way
objects person to beryllium coerced to str
earlier another APIs that anticipate str
paths tin usage them.
possibly Pandas ought to beryllium up to date to judge cases of the summary basal people, os.PathLike
.
quickest most secure manner to bash it is: it volition make if not exists and skip if exists:
from pathlib import way
way("way/with/childs/.../").mkdir(dad and mom=actual, exist_ok=actual)
import os
listing = "./out_dir/subdir1/subdir2"
if not os.way.exists(listing):
os.makedirs(listing)
successful Python three.four you tin besides usage the marque fresh pathlib
module:
from pathlib import way
way = way("/my/listing/filename.txt")
attempt:
if not way.genitor.exists():
way.genitor.mkdir(dad and mom=actual)
but OSError:
# grip mistake; you tin besides drawback circumstantial errors similar
# FileExistsError and truthful connected.
successful Python3, os.makedirs
helps mounting exist_ok
. The default mounting is mendacious
, which means an OSError
volition beryllium raised if the mark listing already exists. By mounting exist_ok
to actual
, OSError
(listing exists) volition beryllium ignored and the listing volition not beryllium created.
os.makedirs(way,exist_ok=actual)
successful Python2, os.makedirs
doesn't activity mounting exist_ok
. You tin usage the attack successful heikki-toivonen's reply:
import os
import errno
def make_sure_path_exists(way):
attempt:
os.makedirs(way)
but OSError arsenic objection:
if objection.errno != errno.EEXIST:
rise
For a 1-liner resolution, you tin usage IPython.utils.way.ensure_dir_exists()
:
from IPython.utils.way import ensure_dir_exists
ensure_dir_exists(dir)
From the documentation: guarantee that a listing exists. If it doesn’t be, attempt to make it and defend in opposition to a contest information if different procedure is doing the aforesaid.
IPython is an delay bundle, not portion of the modular room.
The applicable Python documentation suggests the usage of the EAFP coding kind (simpler to inquire for Forgiveness than approval). This means that the codification
attempt:
os.makedirs(way)
but OSError arsenic objection:
if objection.errno != errno.EEXIST:
rise
other:
mark "\nBE cautious! listing %s already exists." % way
is amended than the alternate
if not os.way.exists(way):
os.makedirs(way)
other:
mark "\nBE cautious! listing %s already exists." % way
The documentation suggests this precisely due to the fact that of the contest information mentioned successful this motion. successful summation, arsenic others notation present, location is a show vantage successful querying erstwhile alternatively of doubly the OS. eventually, the statement positioned guardant, possibly, successful favour of the 2nd codification successful any circumstances --once the developer is aware of the situation the exertion is moving-- tin lone beryllium advocated successful the particular lawsuit that the programme has fit ahead a backstage situation for itself (and another situations of the aforesaid programme).
equal successful that lawsuit, this is a atrocious pattern and tin pb to agelong ineffective debugging. For illustration, the information we fit the permissions for a listing ought to not permission america with the belief permissions are fit appropriately for our functions. A genitor listing might beryllium mounted with another permissions. successful broad, a programme ought to ever activity accurately and the programmer ought to not anticipate 1 circumstantial situation.
one recovered this Q/A last one was puzzled by any of the failures and errors one was getting piece running with directories successful Python. one americium running successful Python three (v.three.5 successful an Anaconda digital situation connected an Arch Linux x86_64 scheme).
see this listing construction:
└── output/ ## dir
├── corpus ## record
├── corpus2/ ## dir
└── subdir/ ## dir
present are my experiments/notes, which supplies clarification:
# ----------------------------------------------------------------------------
# [1] https://stackoverflow.com/questions/273192/however-tin-one-make-a-listing-if-it-does-not-be
import pathlib
""" Notes:
1. see a trailing slash astatine the extremity of the listing way
("methodology 1," beneath).
2. If a subdirectory successful your supposed way matches an current record
with aforesaid sanction, you volition acquire the pursuing mistake:
"NotADirectoryError: [Errno 20] Not a listing:" ...
"""
# Uncomment and attempt all of these "out_dir" paths, singly:
# ----------------------------------------------------------------------------
# methodology 1:
# Re-moving does not overwrite present directories and information; nary errors.
# out_dir = 'output/corpus3' ## nary mistake however nary dir created (lacking tailing /)
# out_dir = 'output/corpus3/' ## plant
# out_dir = 'output/corpus3/doc1' ## nary mistake however nary dir created (lacking tailing /)
# out_dir = 'output/corpus3/doc1/' ## plant
# out_dir = 'output/corpus3/doc1/doc.txt' ## nary mistake however nary record created (os.makedirs creates dir, not information! ;-)
# out_dir = 'output/corpus2/tfidf/' ## fails with "Errno 20" (current record named "corpus2")
# out_dir = 'output/corpus3/tfidf/' ## plant
# out_dir = 'output/corpus3/a/b/c/d/' ## plant
# [2] https://docs.python.org/three/room/os.html#os.makedirs
# Uncomment these to tally "methodology 1":
#listing = os.way.dirname(out_dir)
#os.makedirs(listing, manner=0o777, exist_ok=actual)
# ----------------------------------------------------------------------------
# methodology 2:
# Re-moving does not overwrite present directories and records-data; nary errors.
# out_dir = 'output/corpus3' ## plant
# out_dir = 'output/corpus3/' ## plant
# out_dir = 'output/corpus3/doc1' ## plant
# out_dir = 'output/corpus3/doc1/' ## plant
# out_dir = 'output/corpus3/doc1/doc.txt' ## nary mistake however creates a .../doc.txt./ dir
# out_dir = 'output/corpus2/tfidf/' ## fails with "Errno 20" (present record named "corpus2")
# out_dir = 'output/corpus3/tfidf/' ## plant
# out_dir = 'output/corpus3/a/b/c/d/' ## plant
# Uncomment these to tally "methodology 2":
#import os, errno
#attempt:
# os.makedirs(out_dir)
#but OSError arsenic e:
# if e.errno != errno.EEXIST:
# rise
# ----------------------------------------------------------------------------
decision: successful my sentiment, "technique 2" is much strong.
[1] however tin one safely make a nested listing?
[2] https://docs.python.org/three/room/os.html#os.makedirs
You tin usage mkpath
# make a listing and immoderate lacking ancestor directories.
# If the listing already exists, bash thing.
from distutils.dir_util import mkpath
mkpath("trial")
line that it volition make the ancestor directories arsenic fine.
It plant for Python 2 and three.
successful lawsuit you're penning a record to a adaptable way, you tin usage this connected the record's way to brand certain that the genitor directories are created.
from pathlib import way
path_to_file = way("zero/oregon/much/directories/record.ext")
parent_directory_of_file = path_to_file.genitor
parent_directory_of_file.mkdir(mother and father=actual, exist_ok=actual)
plant equal if path_to_file
is record.ext
(zero directories heavy).
seat pathlib.PurePath.genitor and pathlib.way.mkdir.
wherefore not usage subprocess module if moving connected a device that helps bid
mkdir
with -p
action ?
plant connected python 2.7 and python three.6
from subprocess import telephone
telephone(['mkdir', '-p', 'path1/path2/path3'])
ought to bash the device connected about programs.
successful conditions wherever portability doesn't substance (ex, utilizing docker) the resolution is a cleanable 2 traces. You besides don't person to adhd logic to cheque if directories be oregon not. eventually, it is harmless to re-tally with out immoderate broadside results
If you demand mistake dealing with:
from subprocess import check_call
attempt:
check_call(['mkdir', '-p', 'path1/path2/path3'])
but:
grip...
You person to fit the afloat way earlier creating the listing:
import os,sys,examine
import pathlib
currentdir = os.way.dirname(os.way.abspath(examine.getfile(examine.currentframe())))
your_folder = currentdir + "/" + "your_folder"
if not os.way.exists(your_folder):
pathlib.way(your_folder).mkdir(mother and father=actual, exist_ok=actual)
This plant for maine and hopefully, it volition plant for you arsenic fine
one noticed Heikki Toivonen and A-B-B's solutions and idea of this saltation.
import os
import errno
def make_sure_path_exists(way):
attempt:
os.makedirs(way)
but OSError arsenic objection:
if objection.errno != errno.EEXIST oregon not os.way.isdir(way):
rise
one usage os.way.exists()
, present is a Python three book that tin beryllium utilized to cheque if a listing exists, make 1 if it does not be, and delete it if it does be (if desired).
It prompts customers for enter of the listing and tin beryllium easy modified.
telephone the relation create_dir()
astatine the introduction component of your programme/task.
import os
def create_dir(listing):
if not os.way.exists(listing):
mark('Creating listing '+listing)
os.makedirs(listing)
create_dir('task listing')
usage this bid cheque and make dir
if not os.way.isdir(test_img_dir):
os.mkdir(test_img_dir)
You tin walk the exist_ok=actual parameter to the os.makedirs() relation to suppress the mistake successful lawsuit the listing already exists:
import os
# make listing /way/to/nested/listing if it doesn't already be
os.makedirs('/way/to/nested/listing', exist_ok=actual)
This whitethorn not precisely reply the motion. however one conjecture your existent volition is to make a record and its genitor directories, fixed its contented each successful 1 bid.
You tin bash that with fastcore
delay to pathlib: way.mk_write(information)
from fastcore.utils import way
way('/dir/to/record.txt').mk_write('hullo planet')
seat much successful fastcore documentation